Build Your First Neural Network with TensorFlow in 5 Steps That Won't Fail
-
Miyoko Shimura
- 05 Oct, 2024
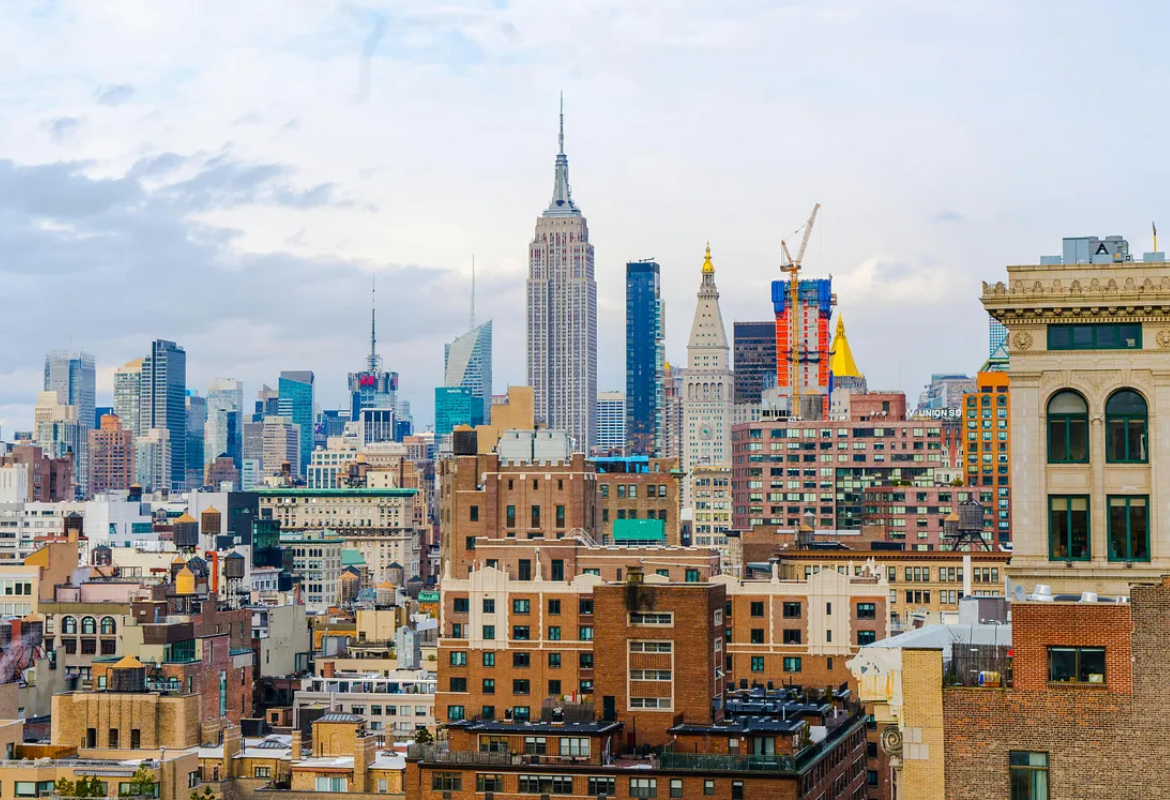
Neural networks are a powerful tool in machine learning, capable of learning complex relationships between inputs and outputs.
In this article, we’ll walk through building a simple neural network using Python and TensorFlow to predict house prices based on the number of bedrooms. With clear process provided, you won’t fail to try your neural network model.
What We Use (Free)
Google Colab is a fantastic tool for running Python code, especially when working with libraries like TensorFlow. It’s free, easy to use, and doesn’t require any installation or setup on your local machine.
- Go to the Google Colab website (https://colab.research.google.com/) and sign in with your Google account.
- Select
New Notebook
to create a new Colab notebook. - Give your notebook a name, like “House Price Prediction”.
- Copy the code from the provided codesnippet and paste it into the each code cell in your Colab notebook on by one.
- Click on the
Run
button (the play icon ▶) for each code cell, or use the keyboard shortcutShift + Enter
to execute the cells one by one. - When prompted, enter the number of rooms for which you’d like to predict the price.
- The notebook will display the predicted price based on your input.
Step 1: Prepare the Dataset
First, create a sample dataset representing the relationship between the number of bedrooms and rent prices.
# Step 1: Prepare the Dataset
import numpy as np
bedrooms = np.array([1.0, 2.0, 3.0, 4.0, 5.0], dtype=float)
rent = np.array([600, 1100, 1600, 2100, 2600], dtype=float)
# Data in thousands
rent_thousands = rent / 1000
By converting the rent to thousands of dollars, we’ve made the numbers more manageable without losing the relationship between variables. This process is called scaling.
In this example, we have a clear correlation: as the number of bedrooms increases, the rent price also increases. Specifically, each additional bedroom adds approximately $500 to the monthly rent.
This dataset is a very simplified representation of the real-world relationship between bedrooms and prices. In practice, you would typically work with larger, more complex datasets that include multiple features (e.g., square footage, location, etc.) and a larger number of samples.
Step 2: Build the Neural Network
Next, build a simple neural network using TensorFlow’s Keras API. Here, we create a sequential model with a single dense layer.
# Step 2: Build the Neural Network
import tensorflow as tf
model = tf.keras.Sequential([
tf.keras.Input(shape=(1,)),
tf.keras.layers.Dense(units=1)
])
tf.keras.Input(shape=(1,))
specifies that the model will accept input data with a single feature. In our case, this corresponds to the number of bedrooms (a single numeric value). The shape(1,)
indicates the input data is a one-dimensional array with a single element (the number of bedrooms).tf.keras.layers.Dense(units=1)
creates a dense layer with one neuron. This means the model will use deep learning to output a single value, which represents the predicted rent price.
Step 3: Compile the Model
Before training the model, we need to configure it with an optimizer and a loss function.
# Step 3: Compile the Model
model.compile(optimizer='sgd', loss='mean_squared_error')
We use stochastic gradient descent (‘sgd’) as the optimizer and mean squared error (‘mean_squared_error’) as the loss function.
Step 4: Train the Model
Now, we can finally train the model using the house price dataset.
# Step 4: Train the Model
model.fit(bedrooms, rent_thousands, epochs=500)
For training, the fit
method takes the input data (bedrooms), the corresponding labels (prices), and the number of training epochs. An epoch is a complete pass through the dataset.
Step 5: Make Predictions
Once your neural network is trained, you can use the model to make predictions. This is the exciting part!
# Step 5: Make Predictions
# Get user input for the number of rooms
num_rooms = int(input("Enter the number of rooms: "))
# Predict the rent
print(f"Predicted rent for {num_rooms} rooms: {model.predict(np.array([num_rooms]), verbose=1).item():.5f}")
The predict
method takes an array of inputs and returns the predicted outputs. We access the first (and only) element of the result to get the predicted price.
Try Out
Let’s predict the price of a house with 6 bedrooms using the formula (0.6 + 5 * 0.5). This gives us an expected price of $3.1k, or $3,100.
The model’s prediction of $3,075 for a 6-bedroom house is quite close to the expected price of $3,100 based on the given formula.
The discrepancy suggests that while the model is performing reasonably well, there is still room for improvement. Some potential reasons for the difference could be limited training data and simplistic model architecture.
This is a fair simple example, but it effectively shows the basic steps in building a neural network!
(Python Full Code)
# Step 1: Prepare the Dataset
import numpy as np
bedrooms = np.array([1.0, 2.0, 3.0, 4.0, 5.0], dtype=float)
rent = np.array([600, 1100, 1600, 2100, 2600], dtype=float)
# Data in thousands
rent_thousands = rent / 1000
# Step 2: Build the Neural Network
import tensorflow as tf
model = tf.keras.Sequential([
tf.keras.Input(shape=(1,)),
tf.keras.layers.Dense(units=1)
])
# Step 3: Compile the Model
model.compile(optimizer='sgd', loss='mean_squared_error')
# Step 4: Train the Model
model.fit(bedrooms, rent_thousands, epochs=500)
# Step 5: Make Predictions
# Get user input for the number of rooms
num_rooms = int(input("Enter the number of rooms: "))
# Predict the rent
print(f"Predicted rent for {num_rooms} rooms: {model.predict(np.array([num_rooms]), verbose=1).item():.5f}")
FAQ
Q1. How do we decide the number of epochs when training a neural network?
To decide the number of epochs when training a neural network, monitor the training and validation loss to check for overfitting. You can also experiment with different epoch values and see how they affect the model’s performance. This way, you can find the best balance for training your model.
Q2. What is the purpose of the optimizer in a neural network?
The optimizer is an important part of a neural network that changes the model’s weights based on gradients calculated during backpropagation.
Its main goal is to minimize the loss function, which shows how far off the predictions are from the actual values, thus improving the model’s performance. Some common optimizers include
- Stochastic Gradient Descent (SGD): Updates the weights based on the gradients from individual training examples.
- Adam: Adjusts the learning rate for each weight based on its past gradients.
- RMSprop: Keeps a moving average of the squared gradients to stabilize the learning rate.
Conclusion
Congratulations! 🎉 You have built a simple neural network to predict house prices based on the number of bedrooms. You prepared a sample dataset, constructed the model using TensorFlow’s Keras API, compiled it with an optimizer and loss function, trained it on our data, and made a prediction.
With more complex datasets and architectures, neural networks can learn intricate patterns and solve challenging problems.
Further Readings
[1] Google Colab: https://colab.research.google.com/
[2] NumPy: https://numpy.org/
[3] TensorFlow Tutorials: https://www.tensorflow.org/tutorials
[4] DeepLearning.AI TensorFlow Developer Professional Certificate (Coursera): https://www.coursera.org/professional-certificates/tensorflow-in-practice